ChatGPTでプログラミング!Vue.JSのクイズアプリを作ろう
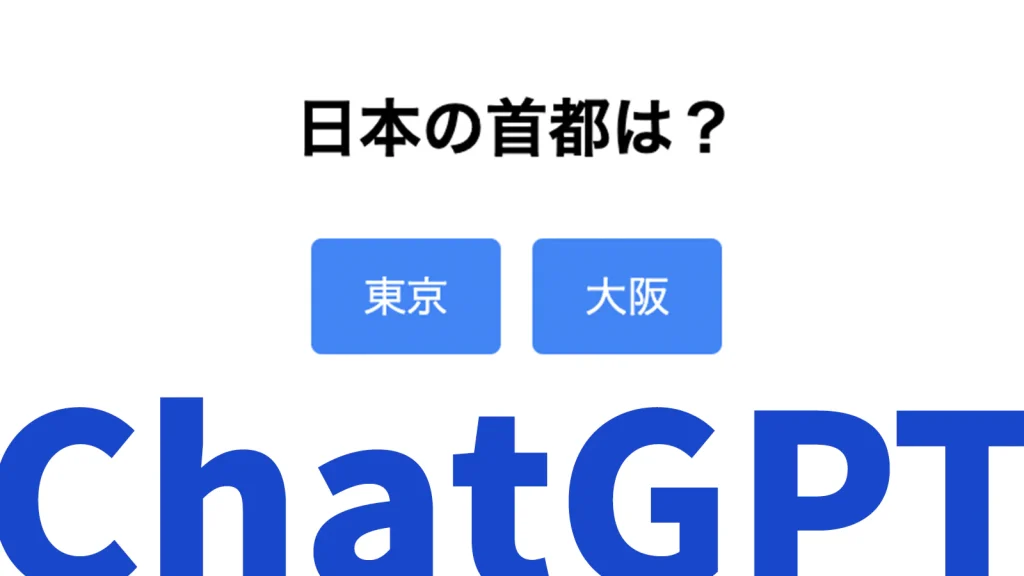
こんにちは。Webデザイナーのショウです。
ChatGPTと対話しながらVue.jsのクイズアプリを作ってみました。作り方の流れを公開します。完成したアプリはこちらです。
→ デモ
今回は無料のGPT3.5ではなくGPT4を使用しています。GPT4は月額$20を支払うと利用できます。3.5でも質問してみましたが、4の方がより精確でバグのないコードを書いてくれる気がします。
ChatGPTに開発の要件を伝える
まずは、要件定義というとやや大げさですが、作りたいアプリの内容をChatGPTに伝えました。
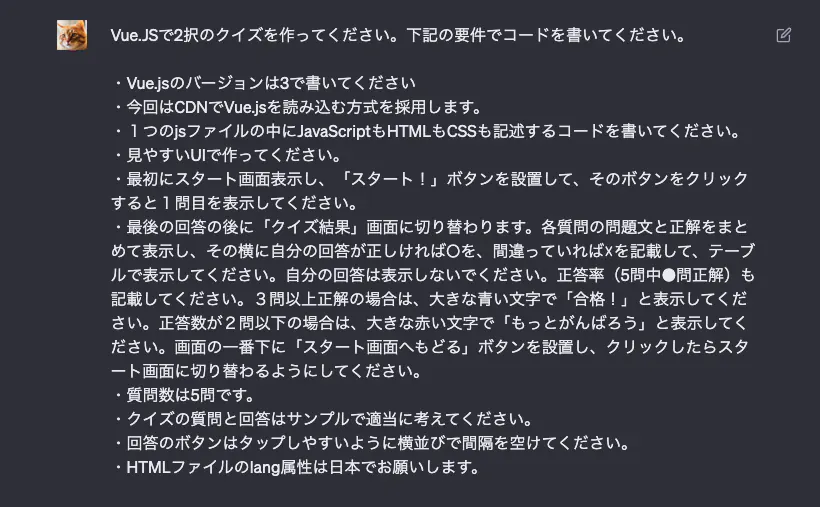
Vue.JSで2択のクイズを作ってください。下記の要件でコードを書いてください。
・Vue.jsのバージョンは3で書いてください
・今回はCDNでVue.jsを読み込む方式を採用します。
・1つのjsファイルの中にJavaScriptもHTMLもCSSも記述するコードを書いてください。
・見やすいUIで作ってください。
・最初にスタート画面表示し、「スタート!」ボタンを設置して、そのボタンをクリックすると1問目を表示してください。
・最後の回答の後に「クイズ結果」画面に切り替わります。各質問の問題文と正解をまとめて表示し、その横に自分の回答が正しければ○を、間違っていれば☓を記載して、テーブルで表示してください。自分の回答は表示しないでください。正答率(5問中●問正解)も記載してください。3問以上正解の場合は、大きな青い文字で「合格!」と表示してください。正答数が2問以下の場合は、大きな赤い文字で「もっとがんばろう」と表示してください。画面の一番下に「スタート画面へもどる」ボタンを設置し、クリックしたらスタート画面に切り替わるようにしてください。
・質問数は5問です。
・クイズの質問と回答はサンプルで適当に考えてください。
・回答のボタンはタップしやすいように横並びで間隔を空けてください。
・HTMLファイルのlang属性は日本でお願いします。
今回はVue.JSはCDN方式で読み込み、1つのHTMLファイルにHTML・CSS・JavaScriptを記述するようにします。さらにコードの難読化や隠蔽はしないためソースコード上で解答が見えてしまうので、このコードを使用する場合は見られてもOKな気軽なクイズを想定しています。
対話開始!
お、書き始めました!
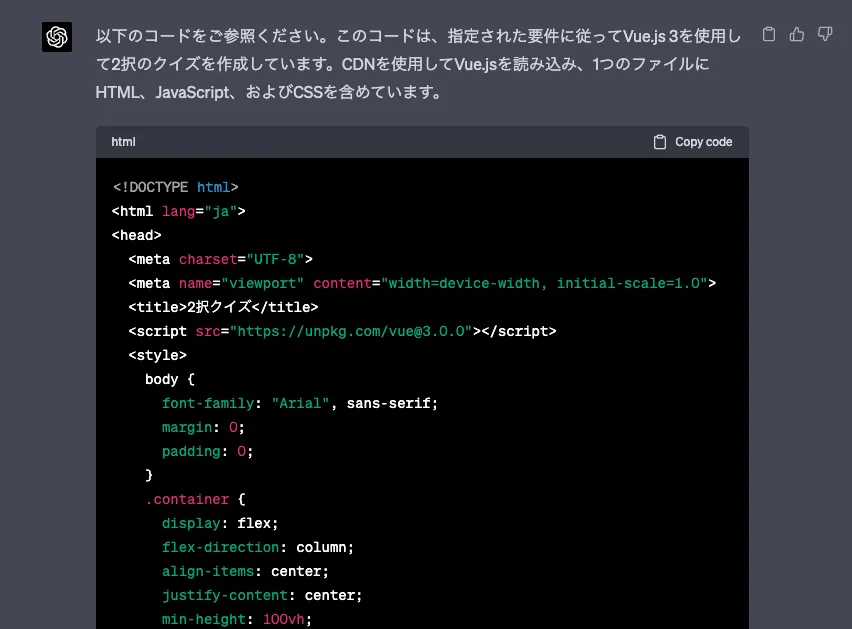
GPT4は以前は回答がゆっくりで遅かったのですが、だいぶ速くなりました。
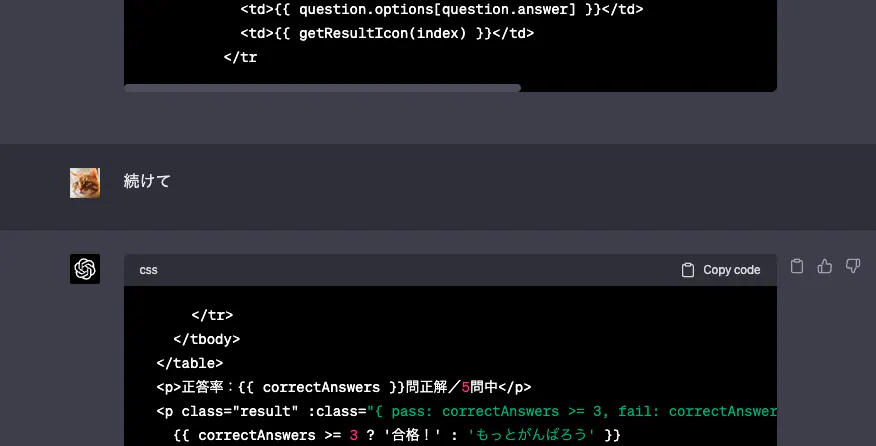
相変わらず途中で止まってしまうので「続けて」と促します。
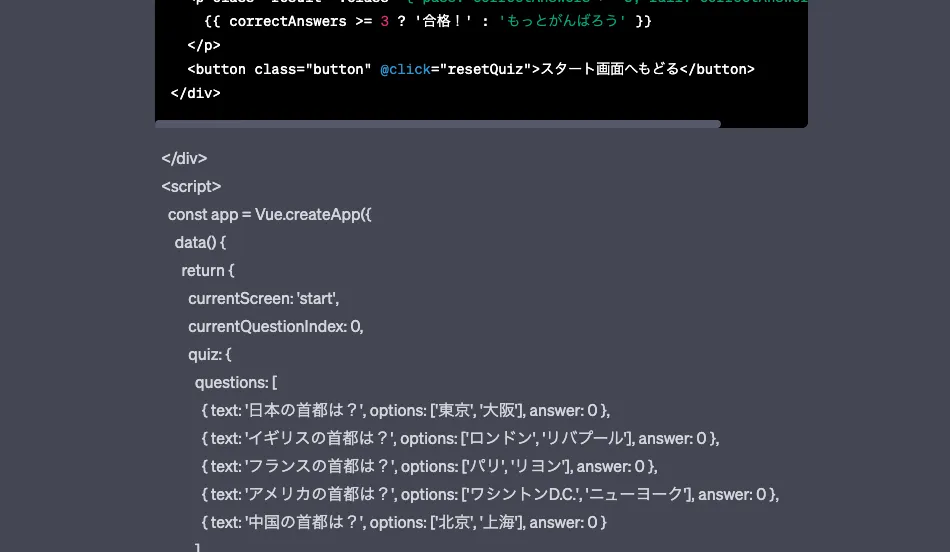
途中、コードブロックからはみ出してテキストで出力しはじめました笑。気にせず見守ります。
完了。だいたい15秒くらいで書いてくれました!
完成したコード
以下がすべてのコードです。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>2択クイズ</title>
<script src="https://unpkg.com/[email protected]"></script>
<style>
body {
font-family: "Arial", sans-serif;
margin: 0;
padding: 0;
}
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
min-height: 100vh;
}
.button-container {
display: flex;
justify-content: space-around;
width: 100%;
max-width: 300px;
margin-top: 1rem;
}
.button {
background-color: #4285f4;
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border-radius: 4px;
}
table {
border-collapse: collapse;
width: 80%;
margin: 1rem auto;
}
th,
td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
color: black;
}
.result {
font-size: 24px;
font-weight: bold;
}
.pass {
color: blue;
}
.fail {
color: red;
}
</style>
</head>
<body>
<div id="app" class="container">
<div v-if="currentScreen === 'start'">
<h1>2択クイズへようこそ!</h1>
<button class="button" @click="startQuiz">スタート!</button>
</div>
<div v-if="currentScreen === 'quiz'">
<h2>{{ currentQuestion.text }}</h2>
<div class="button-container">
<button class="button" v-for="(option, index) in currentQuestion.options" :key="index"
@click="submitAnswer(index)">
{{ option }}
</button>
</div>
</div>
<div v-if="currentScreen === 'results'">
<h1>クイズ結果</h1>
<table>
<thead>
<tr>
<th>問題</th>
<th>正解</th>
<th>結果</th>
</tr>
</thead>
<tbody>
<tr v-for="(question, index) in quiz.questions" :key="index">
<td>{{ question.text }}</td>
<td>{{ question.options[question.answer] }}</td>
<td>{{ getResultIcon(index) }}</td>
</tr>
</tbody>
</table>
<p>正答率:{{ correctAnswers }}問正解/5問中</p>
<p class="result" :class="{ pass: correctAnswers >= 3, fail: correctAnswers <= 2 }">
{{ correctAnswers >= 3 ? '合格!' : 'もっとがんばろう' }}
</p>
<button class="button" @click="resetQuiz">スタート画面へもどる</button>
</div>
</div>
<script>
const app = Vue.createApp({
data() {
return {
currentScreen: 'start',
currentQuestionIndex: 0,
quiz: {
questions: [
{ text: '日本の首都は?', options: ['東京', '大阪'], answer: 0 },
{ text: 'イギリスの首都は?', options: ['ロンドン', 'リバプール'], answer: 0 },
{ text: 'フランスの首都は?', options: ['パリ', 'リヨン'], answer: 0 },
{ text: 'アメリカの首都は?', options: ['ワシントンD.C.', 'ニューヨーク'], answer: 0 },
{ text: '中国の首都は?', options: ['北京', '上海'], answer: 0 }
],
userAnswers: []
}
};
},
computed: {
currentQuestion() {
return this.quiz.questions[this.currentQuestionIndex];
},
correctAnswers() {
return this.quiz.userAnswers.reduce((sum, userAnswer, index) => {
return sum + (userAnswer === this.quiz.questions[index].answer ? 1 : 0);
}, 0);
}
},
methods: {
startQuiz() {
this.currentScreen = 'quiz';
},
submitAnswer(answer) {
this.quiz.userAnswers.push(answer);
if (this.currentQuestionIndex < this.quiz.questions.length - 1) {
this.currentQuestionIndex++;
} else {
this.currentScreen = 'results';
}
},
getResultIcon(index) {
return this.quiz.userAnswers[index] === this.quiz.questions[index].answer ? '◯' : '☓';
},
resetQuiz() {
this.currentScreen = 'start';
this.currentQuestionIndex = 0;
this.quiz.userAnswers = [];
}
}
});
app.mount('#app');
</script>
</body>
</html>
まるっとコピーしてHTMLファイルにペーストします。完成しました!
→ デモ
以下、実演の動画です。※音声は無いので安心して再生してください。
機能としては十分ですね。デザインはCSSを調整したらもっといい感じになりそうです。
まとめ:ChatGPTは小規模なアプリやコンテンツ制作で活躍してくれそう
僕のようなWebデザイナーはプログラミングにはやや弱いので、このようにChatGPTにベースとなるコードを書いてもらえると本当に助かります。ゼロから書くとすごく時間がかかってしまいますからね…。
ただ、たまにバグのあるコードを生成してしまうので要注意です。どこにバグがあるか気づけるくらいのプログラミングの基礎スキルはあった方が良いです。
CDNのVue.jsを使えば、例えばクイズをサイトのページに埋め込んで何か魅力的なコンテンツにしたり、いろんな活用方法がありそうですね。さらに他にも、診断アプリや占いアプリも作れそうです。
大規模なアプリやシステム開発はまだまだ難しそうですが、こういった小規模なアプリやコンテンツ作りにはChatGPTは活躍してくれそうです。